simple AI-powered website generator with django
Django developer 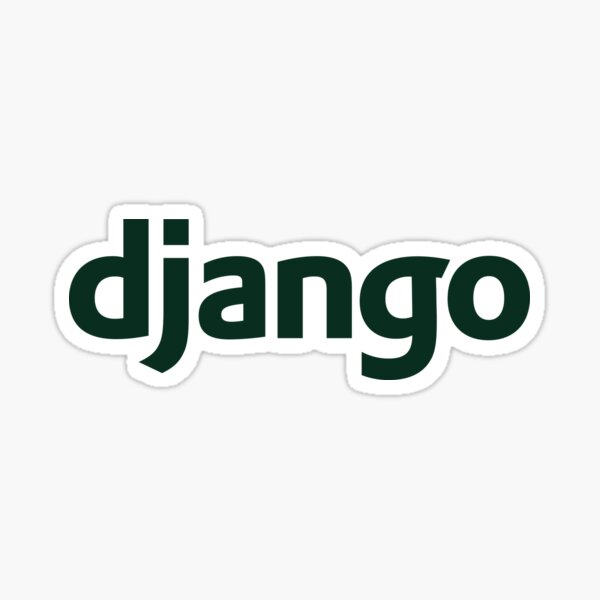
The salary of a Django developer in India can vary depending on several factors such as experience, location, company size, and skillset. Below is an overview of the typical salary ranges for Django developers in India:
1. Entry-Level Django Developer (0-2 years experience)
- Salary Range: ₹3,00,000 - ₹6,00,000 per annum
- Key Skills: Basic understanding of Python, Django, HTML, CSS, JavaScript, relational databases (like PostgreSQL, MySQL), and version control systems (Git).
2. Mid-Level Django Developer (2-5 years experience)
- Salary Range: ₹6,00,000 - ₹12,00,000 per annum
- Key Skills: Proficient in Django and Python, experience with API development, deployment, cloud services (AWS, Azure), advanced database management, and possibly some front-end frameworks (React, Vue.js).
3. Senior Django Developer (5+ years experience)
- Salary Range: ₹12,00,000 - ₹20,00,000+ per annum
- Key Skills: Strong expertise in Django, Python, REST APIs, database optimization, cloud services, Docker, Kubernetes, DevOps practices, and leadership skills. You may also be responsible for mentoring junior developers and managing teams.
4. Freelance or Contract Django Developer
- Hourly Rate: ₹500 - ₹2,000 per hour (depending on the project scope and expertise)
- Project-Based Salary: ₹5,00,000 - ₹15,00,000 for full-scale web applications or ongoing projects
Creating a website using Django with integrated AI tools (like a simple AI-powered website generator) involves several steps. Below is a step-by-step guide to build a website with Django where an AI tool can generate content based on a user’s prompt.
This example uses OpenAI's GPT-4 for content generation, but you can integrate any AI tool you prefer.
Step-by-Step Guide to Create a Django Website with AI Integration
Step 1: Create a Blog Post Model
In your Django app (let's use the generator
app from the previous example), define a model for a blog post. This model can have fields like:
- Title
- Content
- Published Date
- Permalink
- Location
- Search Description (for SEO)
- Create the model in
generator/models.py
:
Step 2: Migrate the Database
Run the following commands to create the database table for the BlogPost
model:
Step 3: Create a Form for Blog Post Submission
If you want to allow users to create posts via a form on your website, create a form for the BlogPost
model.
- Create a form in
generator/forms.py
:
Step 4: Create Views for Creating and Displaying Posts
- Create the view for submitting a post in
generator/views.py
:
Step 5: Set Up URLs for Viewing and Creating Posts
- Add URLs in
generator/urls.py
:
Step 6: Create Templates for Post Submission and Display
Template for creating a post (
generator/templates/create_post.html
):
Template for displaying a post (generator/templates/post_detail.html
):
Step 7: Admin Panel (Optional)
To manage blog posts through the Django admin panel, register the BlogPost
model in generator/admin.py
:
- Add the following to
generator/admin.py
:
Step 8: Testing
Create a superuser to access the Django admin:
Run the server and test the blog post creation:
Comments
Post a Comment